Intro
In this part of the blog series, I want to deploy a new virtual network for my Azure Virtual Desktop environment. I also want to create a network peering between the new network and the one I already deployed named vnet-connectivity-001.
Azure Virtual Desktop network
As I created a virtual network already in the blogs series, I will make a copy of the code for the network and adjust it to fit this new network I am creating. I will copy the three files I have in the “rg-connectivity-network-001” folder and paste them into a new folder called “rg-avd-network-001.”
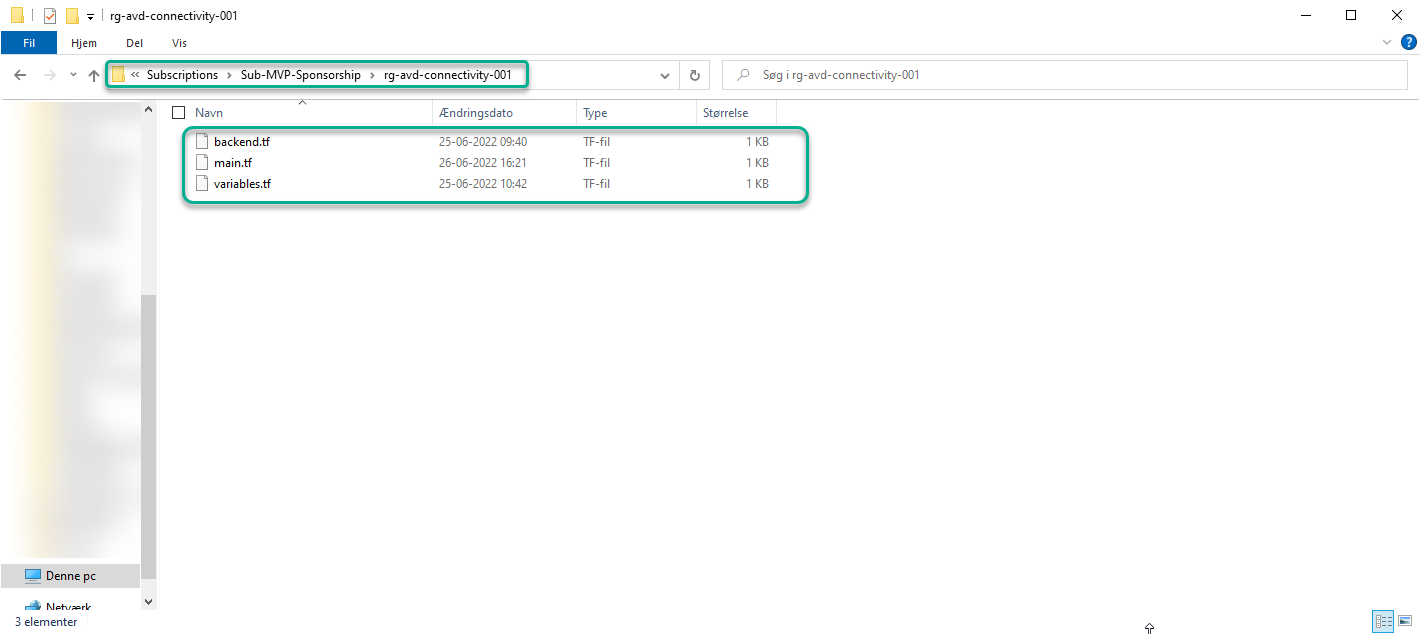
With the files copied, I can change them one by one. I will start with the backend.tf file and ensure that my backend will use another state file. I will name this “GitHub-Terraform-rg-avd-network-001.”
terraform {
required_providers {
azurerm = {
source = "hashicorp/azurerm"
version = "=3.0.0"
}
}
backend "azurerm" {
resource_group_name = "rg-terraform-state-001"
storage_account_name = "cloudninjaterraformstate"
container_name = "tfstate"
key = "GitHub-Terraform-rg-avd-network-001"
}
}
provider "azurerm" {
features {}
}
Next, I will change my variables file to match what I want the new virtual network named and which properties it will have. Notice that I have added a new variable into the file called “RemotevNet,” this is being used to peer the new network to the current one. The new variable contains three values, one for the virtual network name, one for the connection name, and the last for the resource group.
variable "Location" {
type = string
default = "WestEurope"
}
variable "ResourceGroup" {
type = string
default = "rg-avd-network-001"
}
variable "vnet" {
type = any
default = {
"vNetName" = "vnet-avd-001"
"address_space" = ["172.17.0.0/16"]
}
}
variable "Subnets" {
type = any
default = {
"snet-avd-services-001" = {
"name" = "snet-avd-services-001"
"prefix" = ["172.17.0.0/26"]
}
"snet-avd-hostpool-001" = {
"name" = "snet-avd-hostpool-001"
"prefix" = ["172.17.0.64/26"]
}
}
}
variable "RemotevNet" {
default = {
"connectionname" = "AVD-To-Connectivity"
"name" = "vnet-connectivity-001"
"resourcegroup" = "rg-connectivity-network-001"
}
}
The main.tf file is not changed at all compared to the previously created network, and this is what I like about using infrastructure as code. Being able to deploy resources with the same code and only change variables ensures that I have a consistent deployment for my environments.
resource "azurerm_resource_group" "resourcegroup" {
name = var.ResourceGroup
location = var.Location
}
resource "azurerm_virtual_network" "vnet" {
name = var.vnet.vNetName
address_space = var.vnet.address_space
location = azurerm_resource_group.resourcegroup.location
resource_group_name = azurerm_resource_group.resourcegroup.name
}
resource "azurerm_subnet" "subnets" {
for_each = var.Subnets
name = each.value["name"]
resource_group_name = azurerm_resource_group.resourcegroup.name
virtual_network_name = azurerm_virtual_network.vnet.name
address_prefixes = each.value["prefix"]
depends_on = [
azurerm_virtual_network.vnet
]
}
I created a new file, placed it in the same folder, and named it “peering.tf.” I made this new file instead of putting the code inside the main.tf file because creating peerings requires the remote network to exist already. To ensure I can deploy without errors, I add this file after making my virtual networks, so I know they exist.
data "azurerm_virtual_network" "RemotevNet" {
name = var.RemotevNet.name
resource_group_name = var.RemotevNet.resourcegroup
}
resource "azurerm_virtual_network_peering" "AVD-To-Connectivity" {
name = var.RemotevNet.connectionname
resource_group_name = azurerm_resource_group.resourcegroup.name
virtual_network_name = azurerm_virtual_network.vnet.name
remote_virtual_network_id = data.azurerm_virtual_network.RemotevNet.id
}
The image below shows the vNet peering after I deployed the code. Notice that the status says “Initiated,” this is because I haven’t created a peering in the vnet-connectivity-001 virtual network yet.
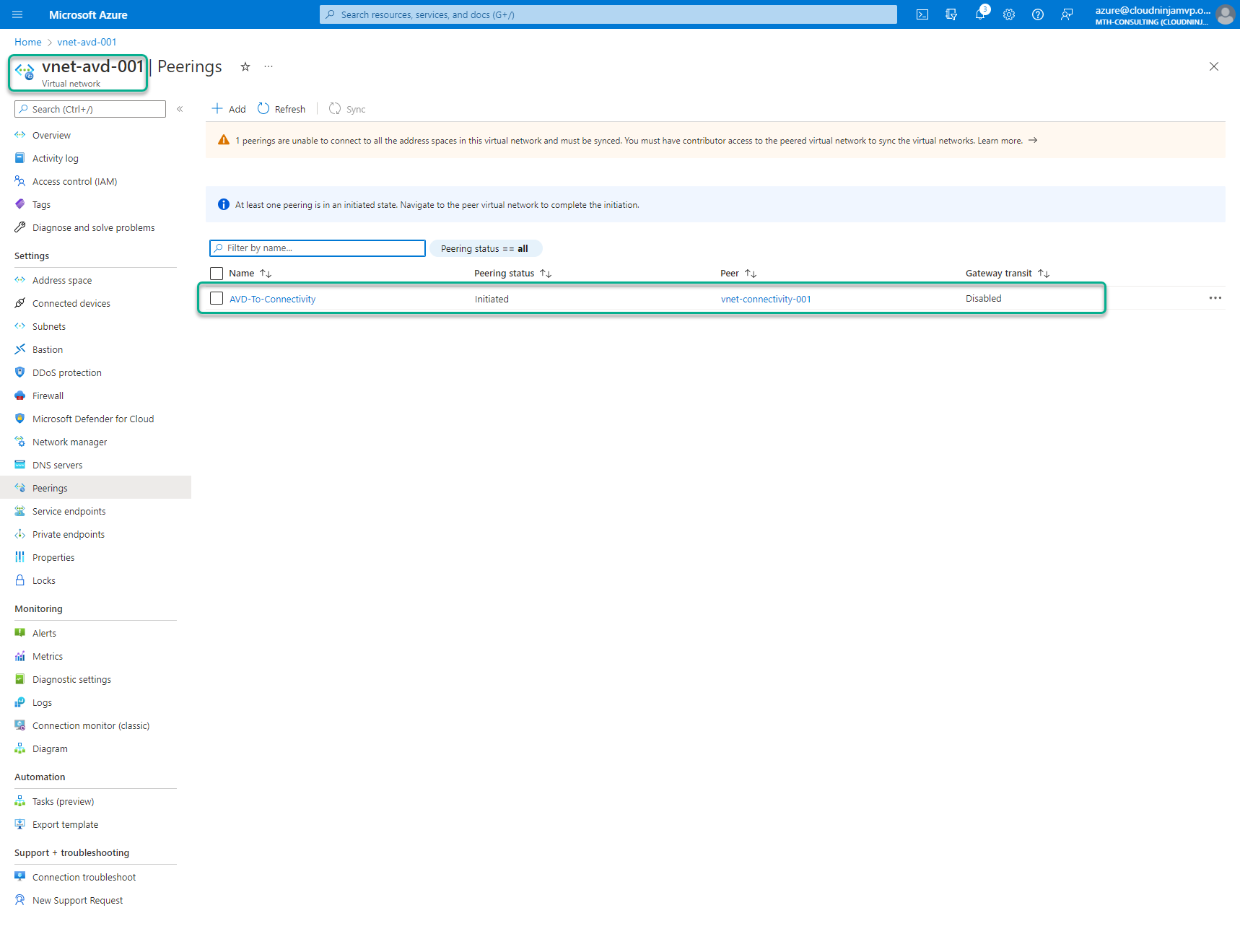
Peering in vnet-connectivity-001
As mentioned in the last section, I must also create a peering from vnet-connectivity-001 to vnet-avd-001. To do this, I made the same peering.tf file in the “rg-connectivity-network-001” folder and added the lines below to the variables.tf file.
variable "RemotevNet" {
default = {
"connectionname" = "Connectivity-To-AVD"
"name" = "vnet-avd-001"
"resourcegroup" = "rg-avd-network-001"
}
}
With the code committed to GitHub, my peering is now complete, and I can verify that in the portal, as shown below.
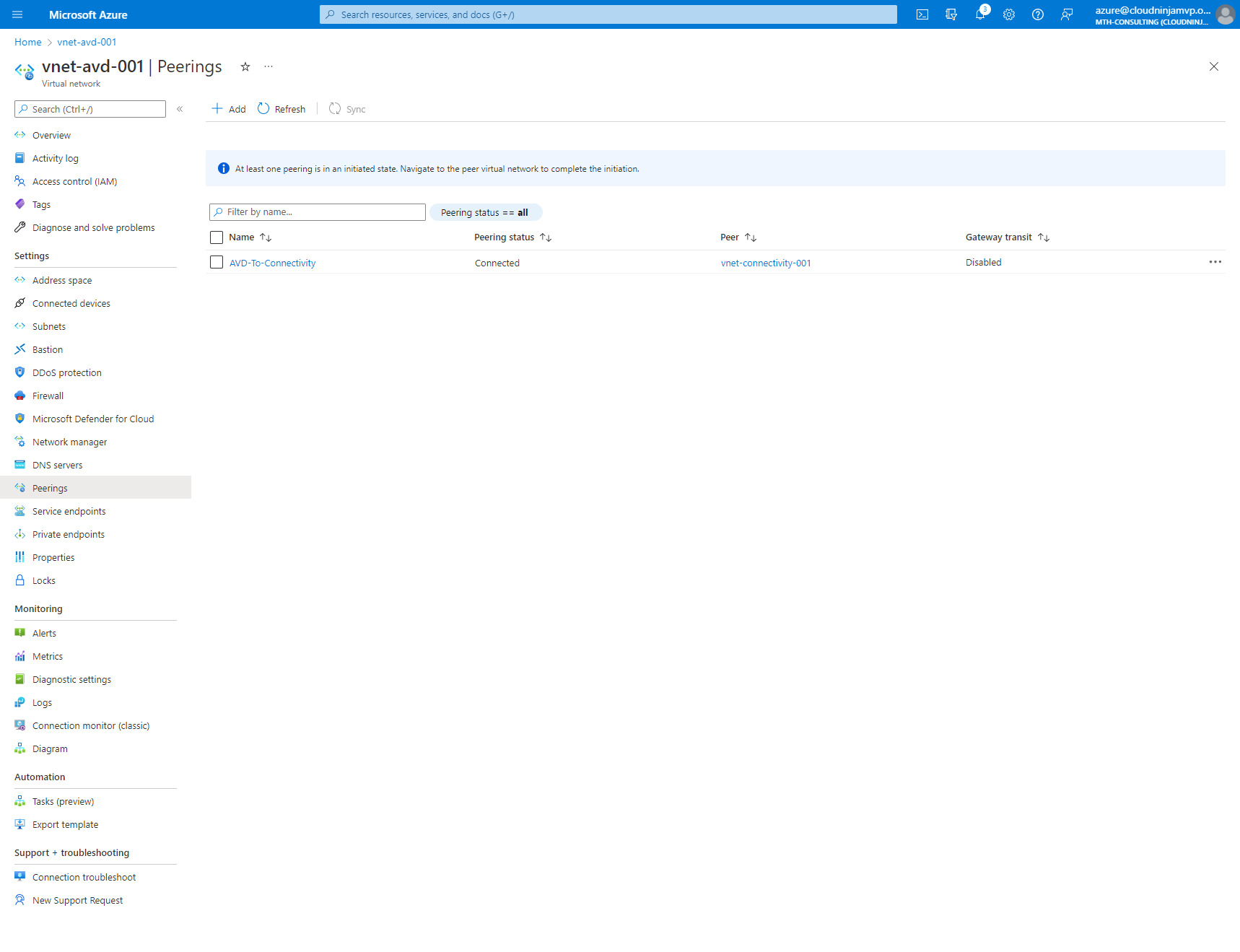
Summary
I can now complete this fourth part of the blog series. I created the virtual network for the upcoming AVD environment and ensured that it has peered with the connectivity network so I could reach this through my VPN connection. I know this has been a short post, but I do think it is an excellent place to end for this time since the next part will be creating the AVD environment backend, and I don’t want to mix those two.
Any feedback is welcome, so reach out on Twitter or LinkedIn, so I can fix any errors or optimize the code I am using.
Links to other parts of the blog series
Part 1: https://www.cloudninja.nu/post/2022/06/github-terraform-azure-part1/
Part 2: https://www.cloudninja.nu/post/2022/06/github-terraform-azure-part2/
Part 3: https://www.cloudninja.nu/post/2022/06/github-terraform-azure-part3/
Part 5: https://www.cloudninja.nu/post/2022/07/github-terraform-azure-part5/
Part 6: https://www.cloudninja.nu/post/2022/07/github-terraform-azure-part6/
Part 7: https://www.cloudninja.nu/post/2022/08/github-terraform-azure-part7/
Part 8: https://www.cloudninja.nu/post/2022/08/github-terraform-azure-part8/
Link for all the code in this post
I have put all the code used in this blog post on my GitHub repository so you can download or fork the repository if you want to.